Blog >
How to create software requirements specification using DRAKON
Request a precise problem statement
Software requirements start with a problem statement. A precise problem statement is a mandatory input for creating requirements. If the client has not provided a problem statement, write one and get the client to approve it.
The problem statement should be a short text, preferably bullet points.
Example:
- Implement password authentication on the website.
- During login, replace the current session with a new one.
- After a successful login, attach the new session to the user record.
- After a successful login, show a personalized start page.
- After a successful login, close all other sessions of the user.
- The system automatically logs the user off after a period without activity.
- If the user has entered an invalid password three times, block the user.
- Make a 3-second pause between password checks.
Images and lists instead of prose
The less plain text there is in the requirements, the better. Instead of writing text, draw diagrams, put together lists and tables.
The requirements will consist of the following documents:
- DRAKON flowcharts that represent the behavior of the software. Most of the complexity of a software system lies in its behavior. Therefore, DRAKON flowcharts will constitute most of the requirements.
- A description of the logical data structure of the system.
- The input and output data formats.
- User interface sketches.
- Acceptance tests.
This article explains how to draw DRAKON flowcharts for software requirements specification. To learn how to write acceptance tests from DRAKON flowcharts, read this: link.
Find the entry points
Look at the program as at a black box that does nothing until it gets a shock from the outside world. There are events in the outside world that set the program in motion. These events are the entry points into the system.
Build a list of entry points. For every entry point, there will be an algorithm that the program will follow to react to the event.
Entry points break up the solid body of the program into pieces following the principle of divide and conquer.
Finding all entry points is crucial. It is not always easy because some entry points are not immediately visible.
Let's take the example with login into a website. The first entry point is "Login with a password." The user presses the "Login" button and authenticates. This one is quite clear.
"The system automatically logs the user off after a period without activity." This statement hides an additional entry point—logout on timeout. Logout on timeout is notable because it will happen in the future.
When the algorithm schedules a future action, that action is also an entry point. In this case, the external shock comes from the alarm clock, not from the user. In spite of this difference, document and test the entry points of postponed actions thoroughly, as usual.
"Postpone logout on timeout" is another entry point. The user postpones his automatic logout with his every action on the website. The problem statement does not mention it explicitly, so this entry point is easy to miss.
Entry points:
- Login with a password
- Logout on timeout
- Postpone logout on timeout
Draw a DRAKON flowchart for every entry point
An external shock comes into an entry point and starts a process. One top-level DRAKON flowchart and possibly several subordinate diagrams describe the algorithm of this process.
Decompose the process into steps—orders and questions
To create a DRAKON diagram of a process, represent the behavior of the system as a series of orders and questions. Follow the principle: one thought—one icon. See a tutorial on drawing DRAKON flowcharts here: link.
Let's take a look at the entry point "Login with a password" from our example.


The happy path
The happy path goes down the left vertical from top to bottom:
- Order: get the username and password from the user.
- Order: find the user by username or email.
- Question: was the user found? Answer: yes.
- Order: check the password.
- Question: did the password check succeed? Answer: yes.
- Order: attach session to the user.
- Order: postpone logout on timeout.
- Order: show the personalized start page.
A less successful scenario goes to the right:
- Question: was the user found? Answer: no.
- Order: show an error message.
- Order: let the user try again after three seconds.
Spare arrows for cycles
Arrows are graphical details that distract the reader and take place on the diagram. DRAKON does not need arrows for the linear flow of events. Time in DRAKON flows down. Since the direction is known, plain lines are sufficient.
Arrows in DRAKON flowcharts show cycles and do not occur often. Hence, arrows make cycles apparent.
Note that the decisions based on the existence of the user and password validity are in the top-level flowchart. Both a wrong username and an incorrect password produce the same visible response from the system: an error message, a 3-second pause, an invitation to try again.
Drop the redundant details
A DRAKON flowchart should show the actions of the system accurately and unambiguously, but without unnecessary technical details and formalisms.
In our example, the Input icon defines that the process gets data from outside—from the user.


The flowchart does not explain how the username and password widgets work, whether they show up simultaneously or one by one. The reader learns only the essence: the user inputs data into the system.
Similarly, the Output icon sends data outside of the process—an error message to the user. The font of the message text and its position on the screen are not relevant on this level of abstraction.
The Pause icon says: the process stops for three seconds, and the user cannot affect the process during that period. "Login with a password" flowchart does not define how to implement the pause. Maybe, the whole page or individual widgets will "freeze" during the pause. These technical details are not necessary to understand the functioning of the system as a whole. If needed, lower level requirements can describe such details.
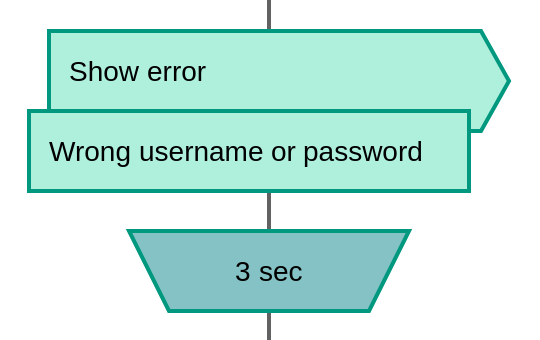
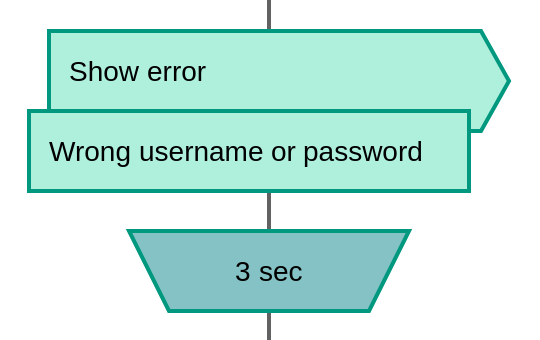
Avoid variables and parameters
Programmers love variables. Programmers assign values to variables and pass variables to function arguments. Variables turn requirements into unreadable code. Variables belong to programs, not to requirements.
Avoid variables in requirements if you can. This advice also applies to passing parameters to subordinate DRAKON flowcharts.
The "Check password" procedure accepts user and password as inputs. The "Check password" flowchart shows those inputs in the Parameters icon.


The Insertion icon in the "Login with a password" flowchart calls the subordinate flowchart "Check password," but the icon text does not include the variables "password" and "user." The Insertion icon contains only the name of the subordinate flowchart—"Check password."
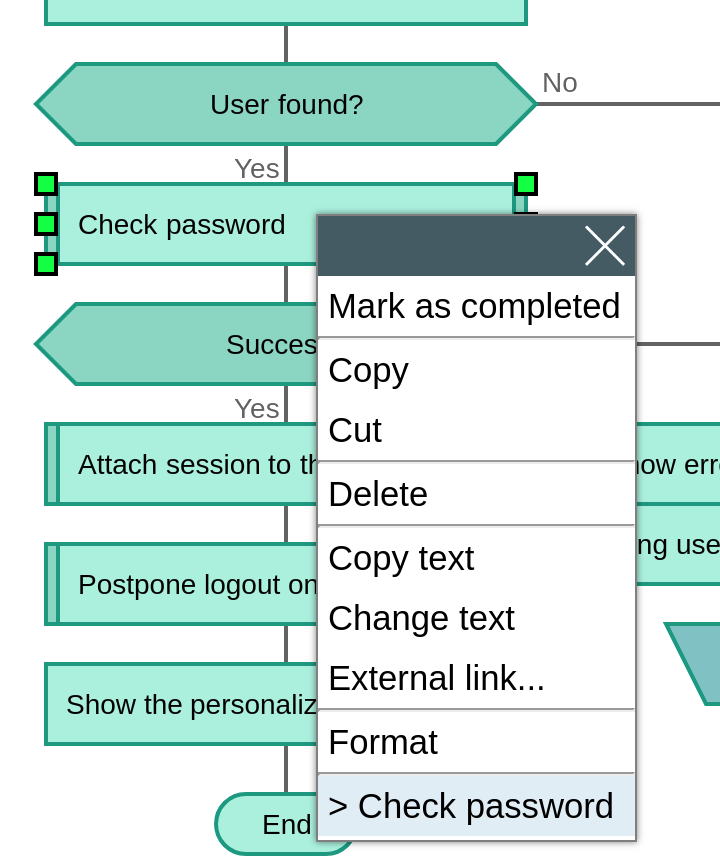
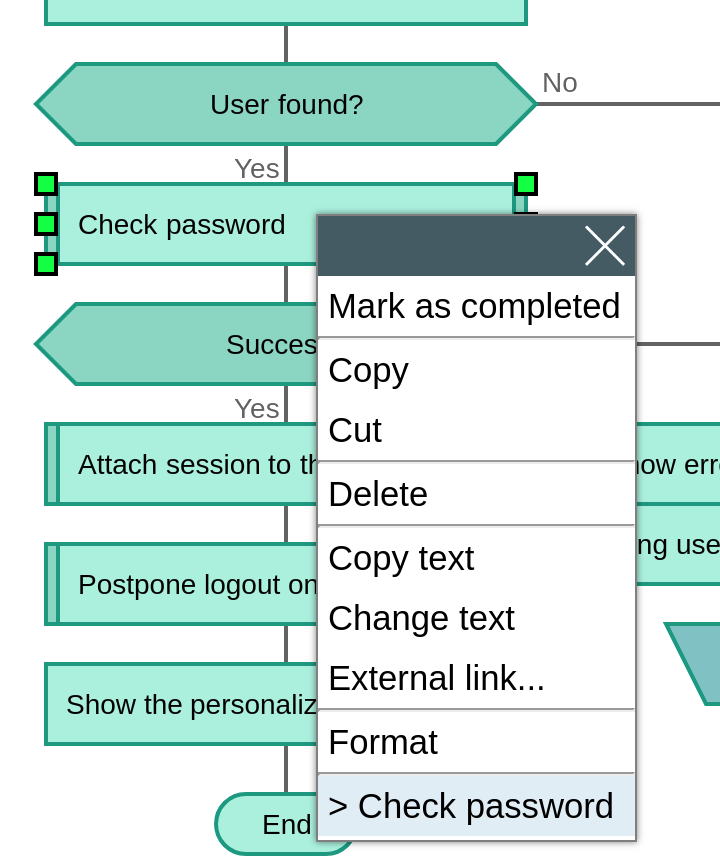
If you right-click that icon, the context menu will show a link to the "Check password" diagram. DrakonHub inserted this link automatically because it found the name of that flowchart in the icon text.
The further to the right, the worse it is
Let us examine procedure "Check password." The happy path in that diagram goes straight down the left vertical, without turning:
- Question: is the user blocked? Answer: no.
- Question: have 3 seconds passed since the last password check? Answer: yes.
- Question: is the password correct? Answer: yes.
- Order: call the password check a success.
Besides the most successful scenario, there are less desirable paths in the diagram. The rule "the further to the right, the worse" prescribes to sort the paths through the flowchart. The less successful the scenario is, the further to the right it should go.
In our example, an incorrect password is worse than the correct one, and blocking the user is worse than giving him one more chance.
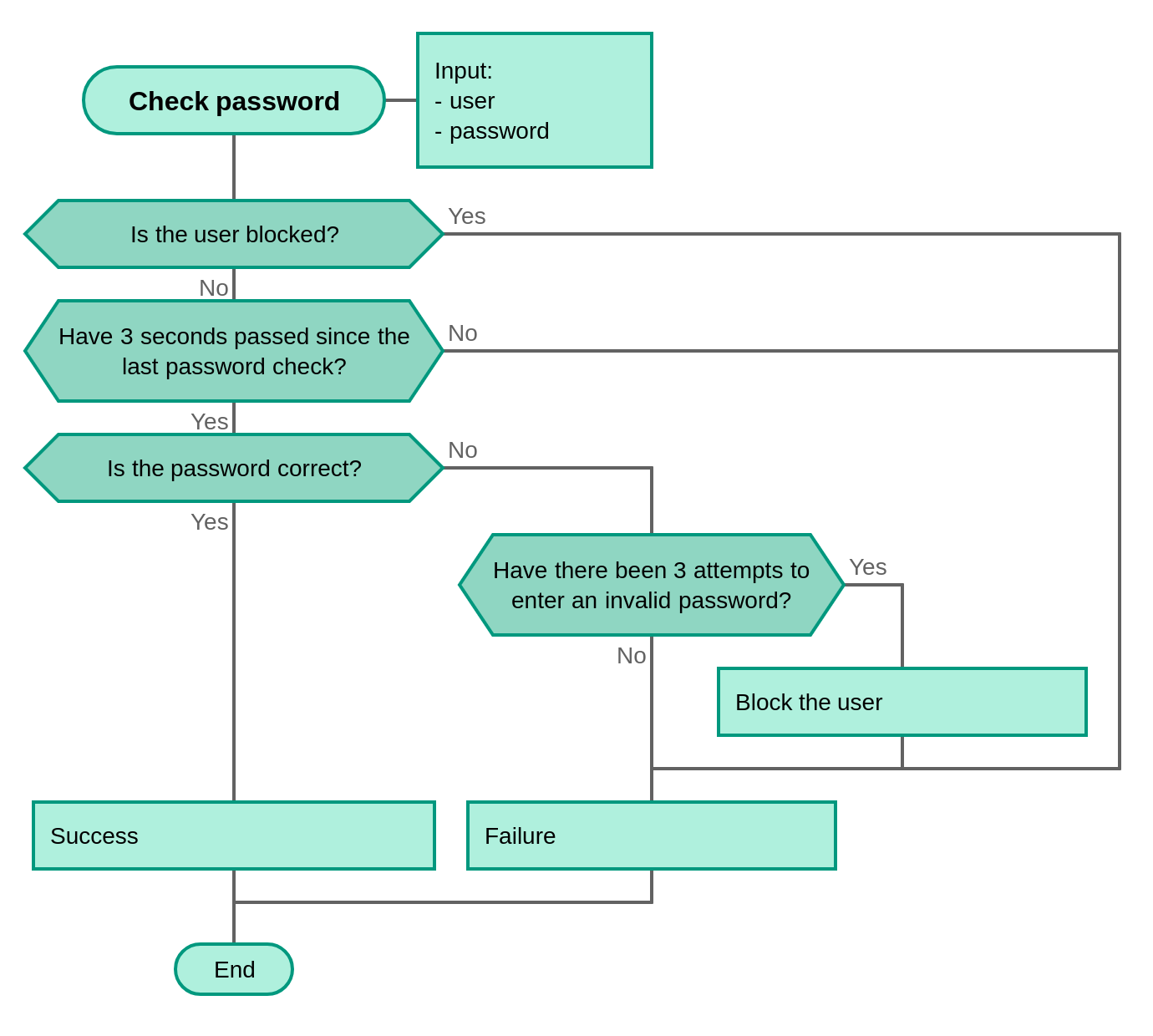
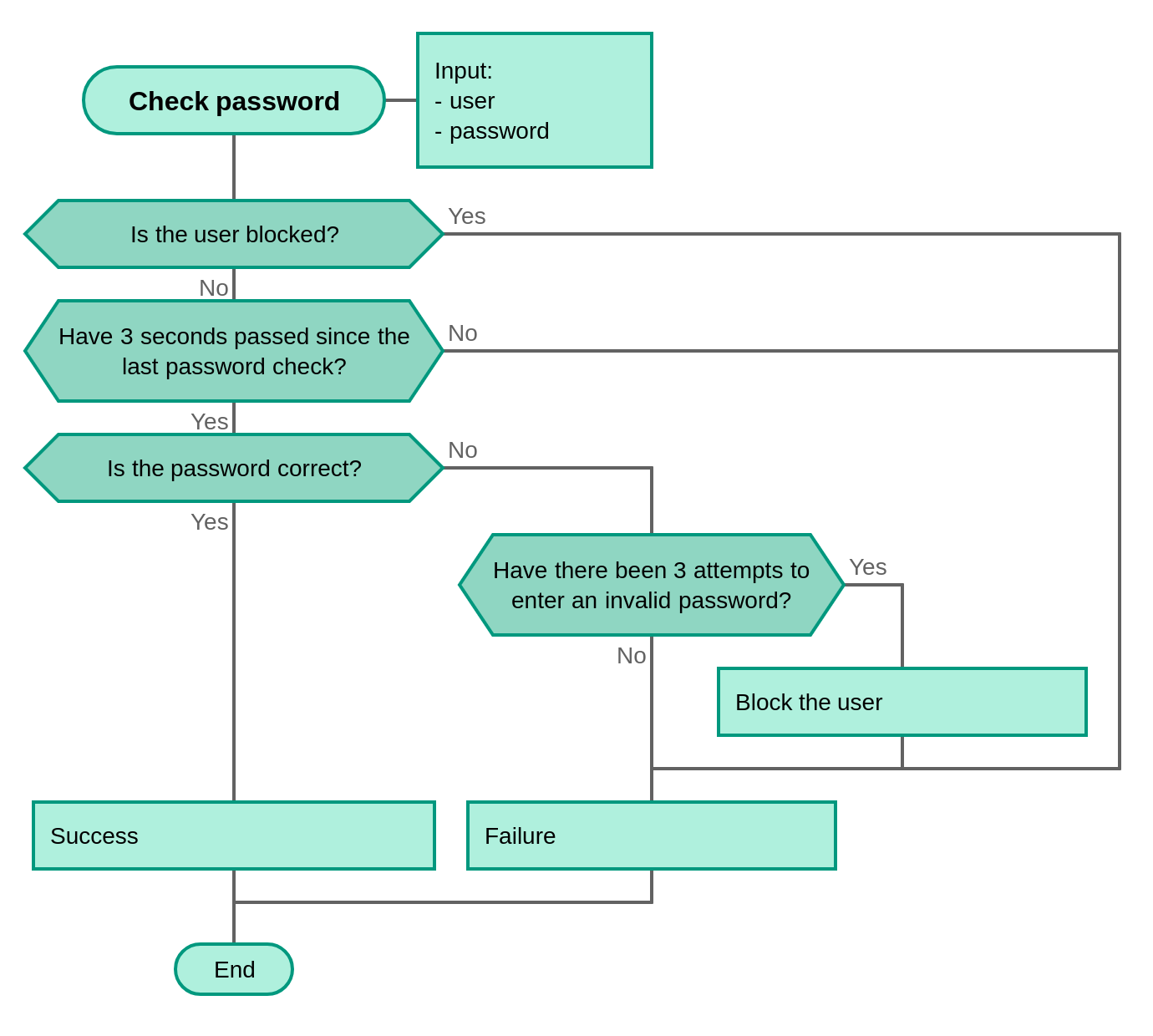
Tell your intention in orders and questions
There are neither variables nor counters for counting unsuccessful login attempts in diagram "Check password." A programmer would find it natural to create such a counter and perform the following actions on it:
- reset the counter in the beginning;
- compare the value of the counter with the number three when the password is incorrect;
- increment the counter after an unsuccessful login.
Do not program in requirements, get rid of variables. Instead of juggling the values of variables, put your intention in the question or order. The intention is the desired result of the step. In our example, the intention is to detect whether the user has made three unsuccessful login attempts. The developers will find a way how to implement this intention.